Exploring the PushbackInputStream Java Method with Examples
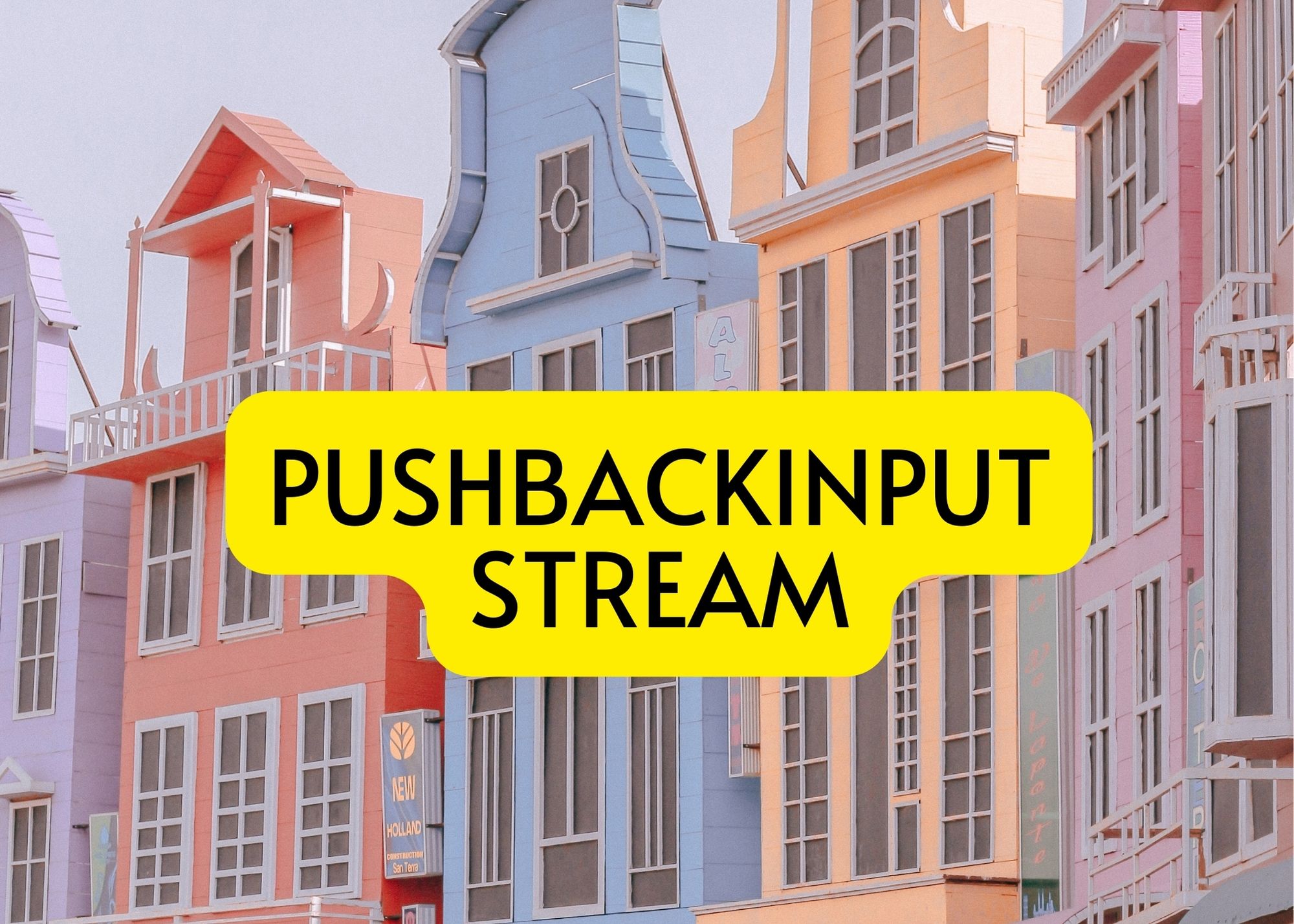
Introduction:
Java’s rich library of classes and methods empowers developers to create efficient and versatile applications. Among these, the PushbackInputStream
class provides a valuable tool for manipulating input streams in a unique way. This article delves into the PushbackInputStream
method, offering a comprehensive understanding of its functionality, use cases, and real-world examples.
Understanding PushbackInputStream:
“The PushbackInputStream
class is a subclass of FilterInputStream
that allows a developer to ""push back"" one or more bytes into the input stream. This mechanism is particularly useful for handling situations where a portion of input data needs to be reconsidered or reprocessed.”
Method Overview:
The primary method of interest in PushbackInputStream
is the unread(int b)
method. This method takes an integer value representing a byte and pushes it back into the stream. It enables developers to manipulate the input stream by providing an opportunity to re-read the byte before proceeding.
Use Cases and Examples:
To illustrate the capabilities of the PushbackInputStream
method, let’s explore a few scenarios where it can be applied effectively.
Example 1: Parenthesis Matching:
Consider a situation where you need to ensure that opening and closing parentheses in an input stream are properly matched. Here’s how the PushbackInputStream
method can help:
"try (PushbackInputStream inputStream = new PushbackInputStream(new FileInputStream(""input.txt""))) {"
int c;
int openedParentheses = 0;
while ((c = inputStream.read()) != -1) {
if (c == '(') {
openedParentheses++;
} else if (c == ')') {
if (openedParentheses > 0) {
openedParentheses--;
} else {
// Push back the ')' character for further processing
inputStream.unread(c);
"System.out.println(""Unmatched closing parenthesis found."");"
}
}
}
if (openedParentheses > 0) {
"System.out.println(""Unmatched opening parentheses found."");"
}
} catch (IOException e) {
e.printStackTrace();
}
Example 2: Peeking at the Next Character:
Imagine a scenario where you want to peek at the next character in the input stream without actually consuming it. This can be achieved using the unread
method:
"try (PushbackInputStream inputStream = new PushbackInputStream(new FileInputStream(""input.txt""))) {"
int c1 = inputStream.read(); // Read the next character
"System.out.println(""Next character: "" + (char) c1);"
inputStream.unread(c1); // Push back the character
int c2 = inputStream.read(); // Read the character again
"System.out.println(""Next character after pushback: "" + (char) c2);"
} catch (IOException e) {
e.printStackTrace();
}
Conclusion:
“The PushbackInputStream
method provides a powerful tool for manipulating input streams in Java. Its ability to ""push back"" bytes into the stream opens up various possibilities for handling, reprocessing, and making decisions based on previously read data. This article has demonstrated two examples showcasing the capabilities of the PushbackInputStream
method. By incorporating this method into your Java applications, you can create more dynamic and adaptable programs that efficiently handle various input scenarios.”