Demystifying the ConditionalOnProperty Annotation in Spring Boot
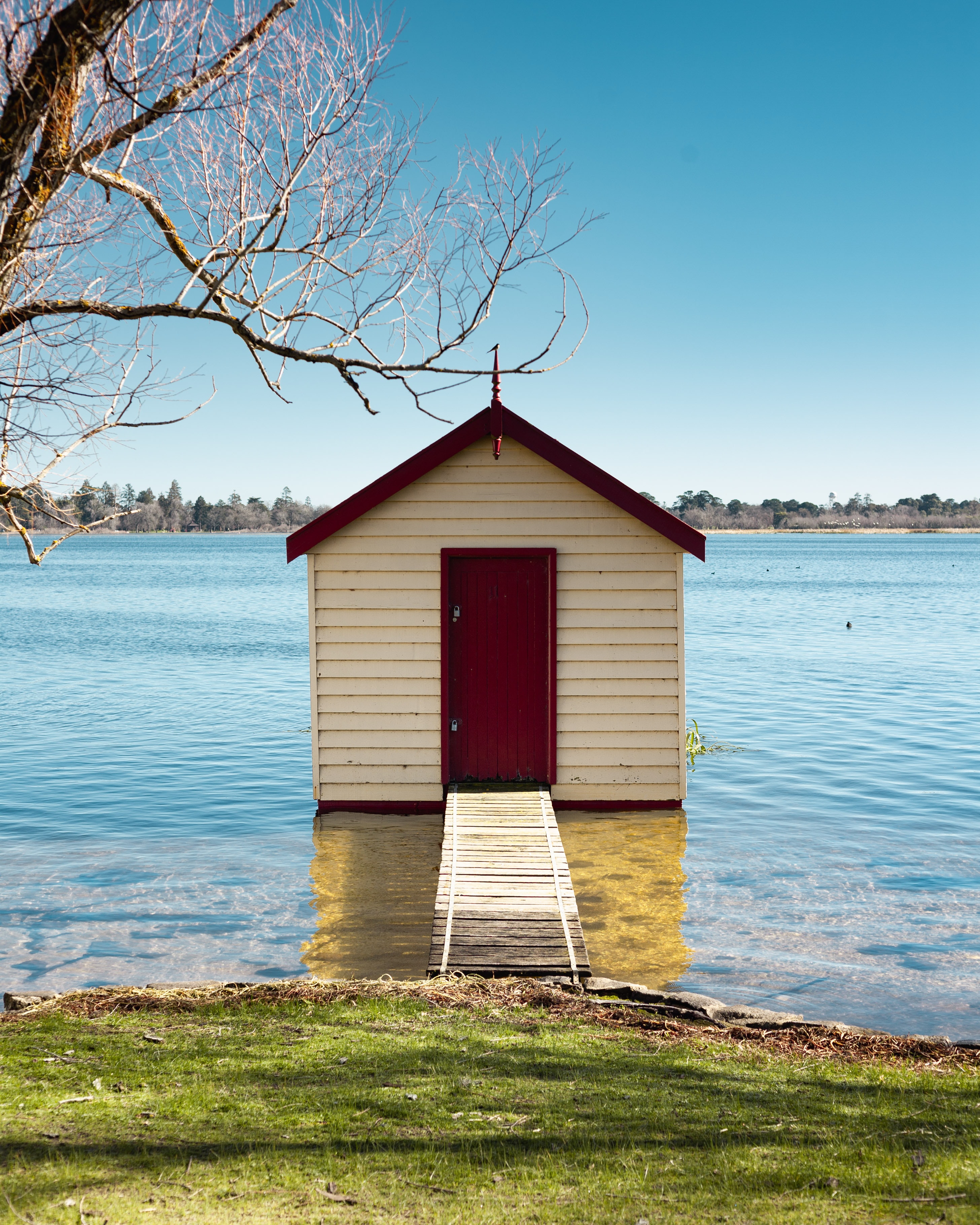
Demystifying the ConditionalOnProperty Annotation in Spring Boot
Introduction:
Spring Boot has revolutionized the world of Java development by providing an elegant framework for creating powerful and scalable applications. Within this ecosystem, the @ConditionalOnProperty
annotation emerges as a dynamic tool that enables developers to configure beans based on specific properties. This article aims to explore the intricacies of the @ConditionalOnProperty
annotation in Spring Boot, shedding light on its functionality, use cases, and practical examples.
Understanding the @ConditionalOnProperty
Annotation:
The @ConditionalOnProperty
annotation is part of Spring Boot’s powerful conditional bean creation mechanism. It allows developers to conditionally create beans based on the values of specific properties in the application’s configuration. This means that a bean will only be instantiated if the defined property conditions are met.
Annotation Attributes:
The @ConditionalOnProperty
annotation comes with various attributes that define the conditions for bean creation. Some of the essential attributes include:
name
: Specifies the name of the property to be evaluated.havingValue
: Specifies the expected value of the property.matchIfMissing
: Determines whether the bean should be created if the property is missing.
Use Cases and Practical Examples:
Let’s dive into some real-world scenarios where the @ConditionalOnProperty
annotation proves to be a valuable asset.
Example 1: Enabling and Disabling Features:
Imagine you have a feature that you want to enable or disable based on a configuration property. Here’s how the @ConditionalOnProperty
annotation can be applied:
@Component
"@ConditionalOnProperty(name = ""feature.enabled"", havingValue = ""true"")"
public class FeatureService {
// Feature-specific code
}
In this example, the FeatureService
bean will only be instantiated if the property feature.enabled
is set to true
.
Example 2: Conditional DataSource Configuration:
Consider a scenario where you have different data sources for different environments. You can use the @ConditionalOnProperty
annotation to conditionally create a DataSource
bean based on the environment:
@Configuration
public class DataSourceConfiguration {
@Bean
"@ConditionalOnProperty(name = ""spring.profiles.active"", havingValue = ""dev"")"
public DataSource devDataSource() {
// Create and configure DataSource for development environment
}
@Bean
"@ConditionalOnProperty(name = ""spring.profiles.active"", havingValue = ""prod"")"
public DataSource prodDataSource() {
// Create and configure DataSource for production environment
}
}
In this case, the appropriate DataSource
bean will be created based on the active profile.
Example 3: External Service Integration:
Suppose you want to integrate an external service only when its configuration is available. The @ConditionalOnProperty
annotation can be used to conditionally create the integration bean:
@Component
"@ConditionalOnProperty(name = ""external-service.enabled"", havingValue = ""true"")"
public class ExternalServiceIntegration {
// Integration logic for the external service
}
Here, the ExternalServiceIntegration
bean will only be instantiated if the property external-service.enabled
is set to true
.
Advanced Use Cases:
Beyond these basic examples, the @ConditionalOnProperty
annotation can be combined with other conditional annotations, such as @Profile
and @ConditionalOnExpression
, to create even more intricate bean creation scenarios.
Conclusion:
The @ConditionalOnProperty
annotation in Spring Boot adds a layer of sophistication to bean creation by allowing developers to conditionally instantiate beans based on property values. Its flexibility makes it a valuable asset for creating dynamic and adaptable applications. This article has provided an in-depth exploration of the @ConditionalOnProperty
annotation, covering its attributes, use cases, and practical examples. By leveraging the power of conditional bean creation, developers can build applications that gracefully adapt to changing requirements and environments, elevating the Spring Boot experience to new heights.