Mastering Software Design Principles with SOLID: Examples and Insights
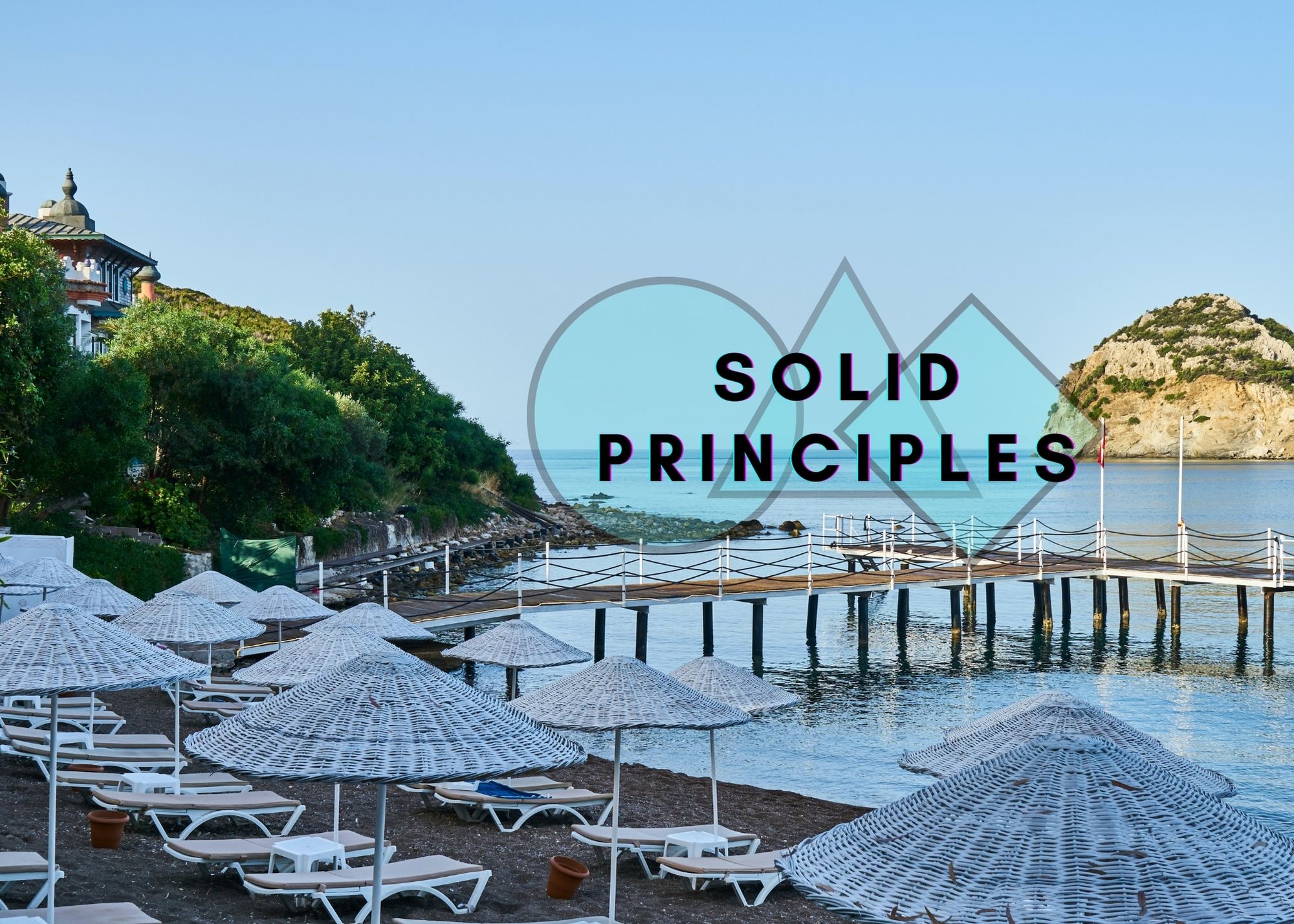
** Mastering Software Design Principles with SOLID: Examples and Insights
Introduction: Software development is a dynamic field that demands scalable, maintainable, and adaptable code. To achieve these goals, developers rely on software design principles that guide them in creating robust and flexible systems. Among these principles, the SOLID principles stand as a foundation for clean and maintainable code. In this article, we will explore each of the five SOLID principles—Single Responsibility Principle, Open-Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle—with real-world examples and insights on their importance in software development.
1. Single Responsibility Principle (SRP): The SRP advocates that a class should have only one reason to change, i.e., it should have a single responsibility. This principle promotes code maintainability by minimizing the impact of changes and promoting modular design.
Example:
Consider a Customer
class that handles both customer information and order processing. Applying SRP, we split it into Customer
and OrderProcessor
classes:
// Before SRP
class Customer {
void processOrder(Order order) {
// Process order logic
}
}
// After SRP
class Customer {
// Customer information related methods
}
class OrderProcessor {
void processOrder(Order order) {
// Process order logic
}
}
2. Open-Closed Principle (OCP): The OCP states that software entities should be open for extension but closed for modification. This principle encourages the use of abstraction and inheritance to accommodate new functionality without altering existing code.
Example:
Consider a Shape
hierarchy with different shapes. Instead of modifying the existing AreaCalculator
class, we create new shape classes that extend Shape
and override the calculateArea
method:
abstract class Shape {
abstract double calculateArea();
}
class Circle extends Shape {
// Implementation of calculateArea for Circle
}
class Rectangle extends Shape {
// Implementation of calculateArea for Rectangle
}
3. Liskov Substitution Principle (LSP): The LSP asserts that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. This principle encourages adhering to the contract defined by the superclass when extending classes.
Example:
If we have a Bird
superclass and subclasses like Sparrow
and Penguin
, the LSP ensures that we can substitute instances of Sparrow
or Penguin
for instances of Bird
without breaking the program’s behavior.
4. Interface Segregation Principle (ISP): The ISP suggests that a class should not be forced to implement interfaces it doesn’t use. Instead of bloating a single interface with multiple methods, segregate interfaces to provide specific functionality to clients.
Example:
Consider an OnlineStore
class that implements an Order
interface. If Order
interface includes shipping methods, it violates ISP for OnlineStore
. Segregate the interface into Order
and Shipping
interfaces:
interface Order {
void placeOrder();
}
interface Shipping {
void shipOrder();
}
class OnlineStore implements Order {
// Implementation of placeOrder
}
class ShippingService implements Shipping {
// Implementation of shipOrder
}
5. Dependency Inversion Principle (DIP): The DIP advocates that high-level modules should not depend on low-level modules; both should depend on abstractions. This principle promotes the use of interfaces to decouple components, allowing for easier testing and future changes.
Example:
Consider a NotificationService
that directly depends on a MessageSender
. Applying DIP, we introduce an interface MessageSender
and let NotificationService
depend on the abstraction:
interface MessageSender {
void sendMessage(String message);
}
class EmailSender implements MessageSender {
// Implementation of sendMessage for email
}
class NotificationService {
private MessageSender sender;
NotificationService(MessageSender sender) {
this.sender = sender;
}
void sendNotification(String message) {
sender.sendMessage(message);
}
}
Conclusion: The SOLID principles provide a guiding light for writing clean, maintainable, and flexible code. By adhering to these principles, developers ensure that their software systems remain adaptable to changing requirements, easier to maintain, and less prone to bugs. Incorporating SOLID principles into your development practices empowers you to create robust software that stands the test of time, fostering a culture of excellence and innovation in software engineering.